Vue2 - 23-03-18
数据绑定
缩写:
v-model:value
=> v-model
v-bind
=> :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>数据绑定</title> <script type="text/javascript" src="../resources/js/vue.js"></script> </head> <body> <div id="root"> 单向数据绑定:<input type="text" v-bind:value="name"><br> 双向数据绑定:<input type="text" v-model:value="name"> <!-- v-model只能应用于表单类元素,即输入类,要有value值 --> <!-- <h2 v-model:x="name">你好</h2> --> <hr> 单向数据绑定:<input type="text" :value="name"><br> 双向数据绑定:<input type="text" v-model="name"> </div> </body> <script type="text/javascript"> Vue.config.productionTip = false; new Vue({ el: '#root', data: { name:'Linzepore', hello:'hello' } }) </script> </html>
|
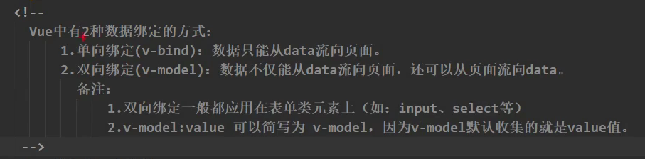
el与data
el跟data都有两种写法,其中data的尤为重要
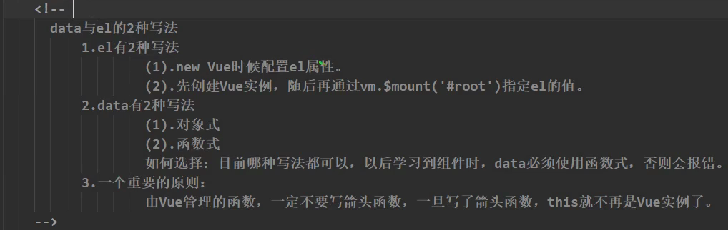
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>el与data区别</title> <script src="../resources/js/vue.js"></script> </head> <body> <div id="root"> <h1>Hello, {{name}}</h1> </div> <script type="text/javascript"> Vue.config.productionTip = false; /*const v = new Vue({ // el: '#root', 第一种写法 data:{ name:'Linzepore' } }) console.log(v) // v.$mount('#root') setTimeout(() => v.$mount('#root'),//第二种写法 1000 )*/ //data的第一种写法:对象式 new Vue({ el:'#root', /*data:{ name:'Linzepore' }*/ //第二种写法:函数式 data:function() { return { name:'Linzepore' } } /*简写 data(){ return { name:'Linzepore' } } */ }) </script> </body> </html>
|
MVVM模型
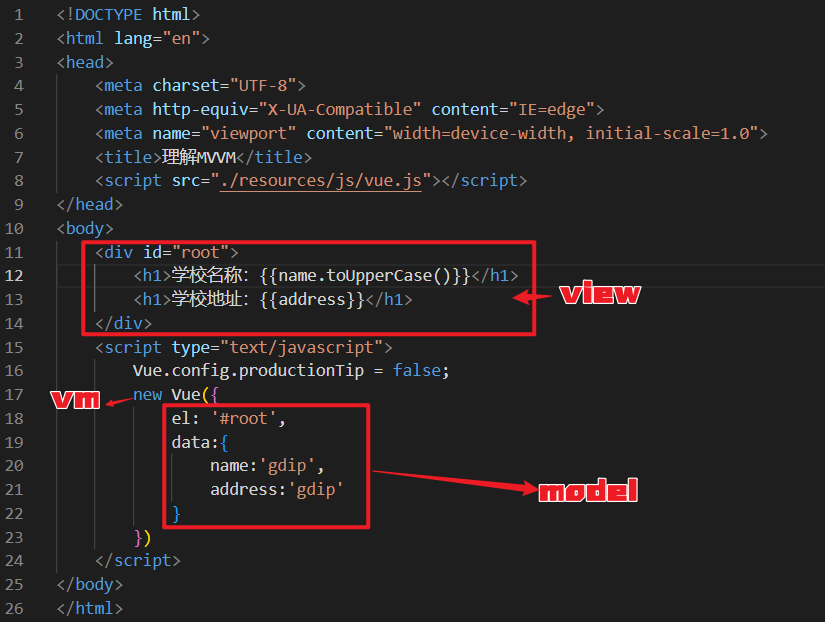
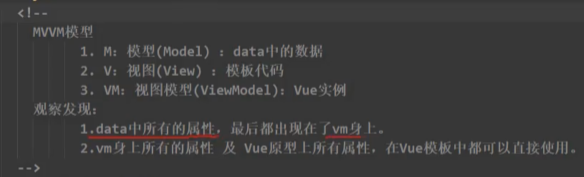
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>理解MVVM</title> <script src="../resources/js/vue.js"></script> </head> <body> <div id="root"> <h1>学校名称:{{name.toUpperCase()}}</h1> <h1>学校地址:{{address}}</h1> <h1>测试一下:{{1+1}}</h1> <h1>测试两下:{{$options}}</h1> <h1>测试三下:{{$emit}}</h1> <h1>测试四下:{{_c}}</h1> </div> <script type="text/javascript"> Vue.config.productionTip = false; const vm = new Vue({ el: '#root', data:{ name:'gdip', address:'gdip' } }) console.log(vm); </script> </body> </html>
|
数据代理前置内容
视频->HERE
关键:通过getter跟setter,可以修改a影响b
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>数据代理回顾</title> </head> <body> <script type="text/javascript"> let number; let person = { name: '张三', sex: '男', //age: number 一次性赋值而已 } console.log(person); console.log(Object.keys(person)); Object.defineProperty(person,'age',{ value:18, enumerable:true, writable:true, configurable:true,
get:function(){ return number; }, set(value) { console.log("有人修改了age,值为"+value); number = value; } }) console.log(person); console.log(Object.keys(person)); </script> </body> </html>
|
何为数据代理
可以给B添加一个属性x,修改B的x会影响A的x
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>何为数据代理</title> <script src="../resources/js/vue.js"></script> </head> <body> <div id="root">
</div> </body> <script type="text/javascript"> Vue.config.productionTip = false; let obj1 = { x : 'xx' } let obj2 = { y : 'yy' } Object.defineProperty(obj2, 'x',{ get:function(){ return obj1.x; }, set:function(value) { obj1.x = value; } }) </script> </html>
|
如图演示:
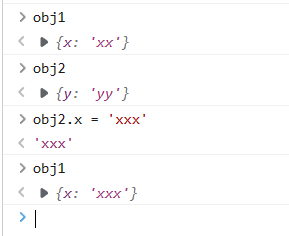
Vue中的数据代理
1 2 3 4 5 6 7
| const vm = new Vue({ el: '#root', data:{ name: 'gdip', address: 'foshan' } })
|
上面的操作中Vue实例中的data=>vm._data=>数据代理转换成属性
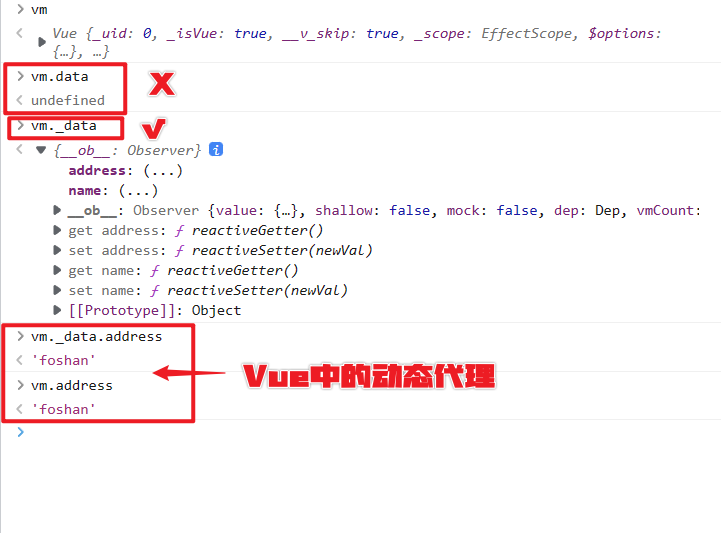
也就是说,vue中的数据代理是如下图显示的过程
事件处理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> <script src="../resources/js/vue.js"></script> </head> <body> <div id="root"> <h2>欢迎来到{{name}}学习</h2> <button v-on:click="showInfo1">点我提醒信息1</button> <!-- 简写 --> <button @click="showInfo1">点我提醒信息1</button> <!-- 传信息 --> <button @click="showInfo2(666,$event)">点我提醒信息2</button> </div> <script type="text/javascript"> Vue.config.productionTip = false; new Vue({ el: '#root', data:{ name:'gdip', }, methods:{ showInfo1(event){ // console.log(event.target.innerText); // console.log(this);此处的this为vm本身 alert('同学你好!'); }, showInfo2(number,event){ console.log(event);//event非必须 console.log(number); alert('同学你好!!'); } } }) </script> </body> </html>
|
1 2 3 4
| <!-- 简写 --> <button @click="showInfo1">点我提醒信息1</button> <!-- 传信息 --> <button @click="showInfo2(666,$event)">点我提醒信息2</button>
|
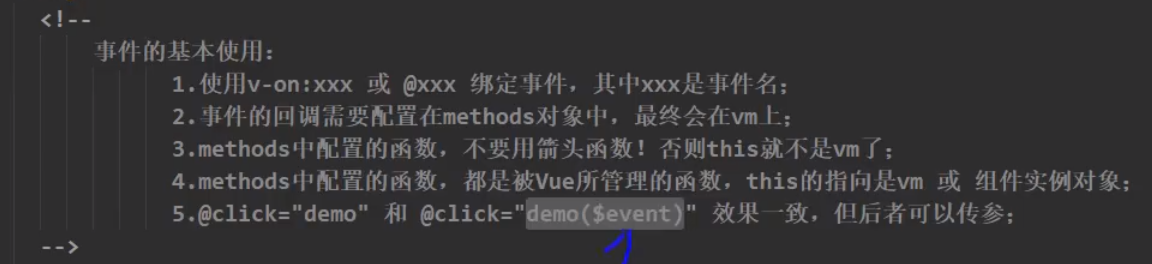
Vue中的事件修饰符
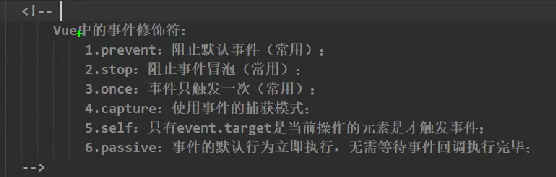
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> <script src="../resources/js/vue.js"></script> <style> .box_fa { padding: 5px; background-color: aquamarine; } .box_ch{ background-color: orange; } .list { width: 200px; height: 200px; background-color: peru; overflow: auto; } .list li { height: 100px; } </style> </head> <body> <div id="root"> <h2>欢迎来到{{name}}的博客</h2> <!-- 阻止默认事件 --> <a href="http://blog.zepo.re" @click="showInfo">会跳转链接</a> <a href="http://blog.zepo.re" @click.prevent="showInfo">点我提醒信息但是不会跳转</a> <!-- 阻止事件冒泡 --> <div @click="showInfo"> <button @click="showInfo">未使用会冒泡</button> </div> <div @click.stop="showInfo"> <button @click.stop="showInfo">修饰了stop不会冒泡</button> </div> <!-- 事件只会触发一次 --> <button @click.once="showInfo">事件只会触发一次</button> <hr> <!-- 事件的捕获阶段由外往内,冒泡阶段由内往外(默认) --> <div class="box_fa" @click="showMsg('father')"> div1 <div class="box_ch" @click="showMsg('child')">div2</div> </div><!-- 点击child,会从内向外冒泡 --> <!-- 只有event.target是当前操作的元素时才触发事件,也就是说如果在父容器使用了.self也能阻止冒泡 --> <div @click="showSelf"> <button @click="showSelf">虽然触发了两次,但是由谁触发的呢,点点试试</button> </div> <div @click.self="showInfo" > <button @click="showInfo">由父容器来进行阻止冒泡</button> </div> <!-- 事件的默认行为立即执行,无需等待事件回调执行完毕 --> <ul @wheel.passive="demo" class="list"><!--先滚动不管回调--> <li>1</li> <li>2</li> <li>3</li> <li>4</li> </ul> </div> <script type="text/javascript"> Vue.config.productionTip = false; new Vue({ el: '#root', data:{ name:"Linzepore" }, methods:{ showInfo(e) { // e.preventDefault() == 属性名.prevent alert('Hello') }, showMsg(msg) { alert(msg); }, showSelf(e) { alert(e.target) }, demo() { for(let i = 0; i < 100000; i++) { console.log('#'); } console.log('累坏了'); } } }) </script> </body> </html>
|
常用的只有三个
1 2 3 4 5 6 7 8 9 10 11 12
| <!-- 阻止默认事件 --> <a href="http://blog.zepo.re" @click="showInfo">会跳转链接</a> <a href="http://blog.zepo.re" @click.prevent="showInfo">点我提醒信息但是不会跳转</a> <!-- 阻止事件冒泡 --> <div @click="showInfo"> <button @click="showInfo">未使用会冒泡</button> </div> <div @click.stop="showInfo"> <button @click.stop="showInfo">修饰了stop不会冒泡</button> </div> <!-- 事件只会触发一次 --> <button @click.once="showInfo">事件只会触发一次</button>
|
修饰符可以连续写
1 2 3
| <div @click.stop="showInfo"> <button @click.stop.prevent="showInfo">修饰了stop不会冒泡</button> </div>
|
键盘事件、按键别名
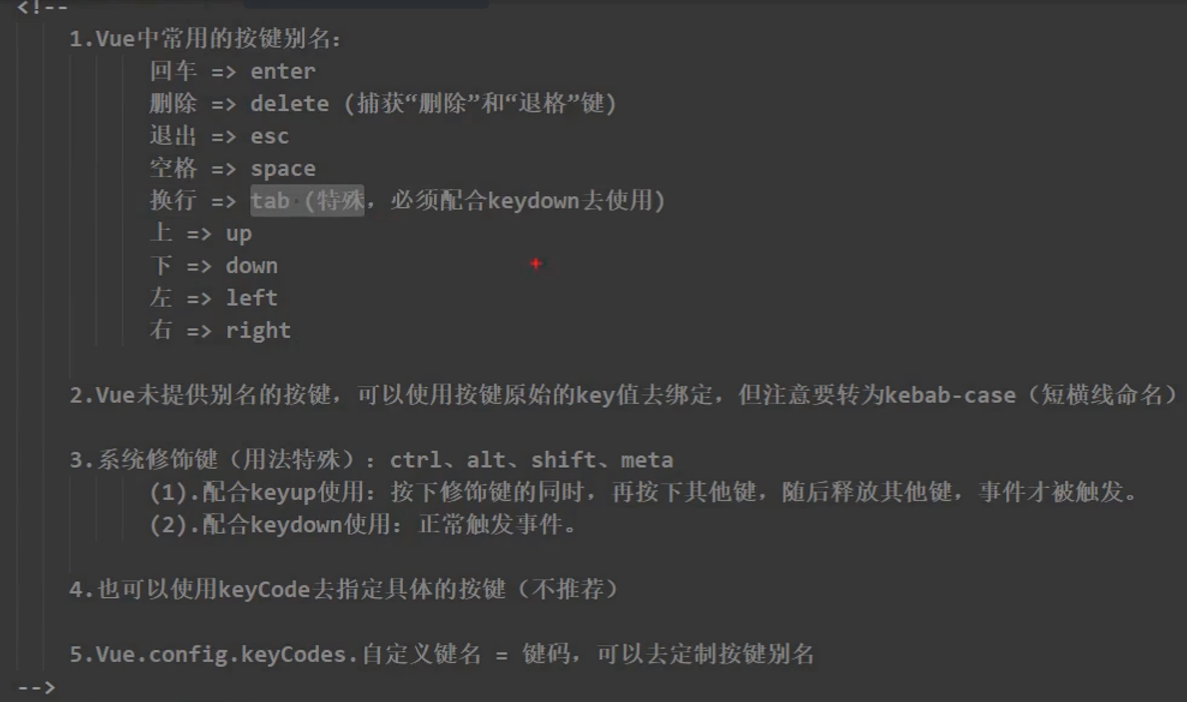
例外
TAB
键会移除焦点,所以要用keyDown
CapsLock
要写成caps-lock
- 系统修饰键
Ctrl
、alt
、shift
、meta
配合keydown可以,但是配合keyup的话要按下并释放其他键之后才会触发1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> <script src="../resources/js/vue.js"></script> </head> <body> <div id="root"> <h2>欢迎来到{{name}}的博客</h2> <input type="text" placeholder="按下回车提醒输入" @keyup.caps-lock="showInfo"> </div> <script type="text/javascript"> Vue.config.productionTip = false; Vue.config.keyCodes.huiche = 13;//定义了一个别名 new Vue({ el: '#root', data:{ name: 'Linzepore' }, methods: { showInfo(e) { // console.log(e.keyCode); // console.log(e.key);//获取真实按键名称 // if(e.keyCode!=13) return//等同于.13 console.log(e.target.value); } } }) </script> </body> </html>
|
也可以连续着来写1
| <input type="text" placeholder="按下回车提醒输入" @keyup.ctrl.y="showInfo">
|