Vue2 - 23-03-31
几种常见的前后端发送接收方式
- XHR
- JQuery
- axios (鱿鱼须推荐)
- fetch
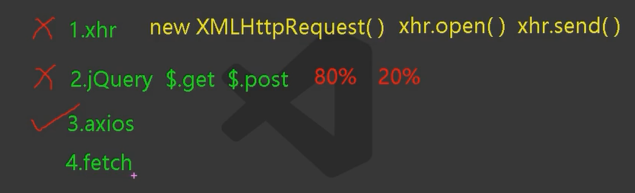
Axios
axios配合Vue使用,简单的get请求就是
import axios from 'axios'
axios.get(xxx).then(func1,func2,...)
代理服务器接收跨域请求
使用代理服务器进行跨域请求有两种常见的方式:
Vue-cli配置代理服务器
vue-cli中通过 vue.config.js
中的 devServer.proxy
选项来配置,将 API 请求代理到 API 服务器
vue-cli文档->【HERE】
单个代理:
1 2 3 4 5
| module.exports = { devServer: { proxy: 'http://localhost:4000' } }
|
不同路径多个代理:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| odule.exports = { devServer: { proxy: { '/api': { target: '<url>', ws: true, changeOrigin: true }, '/foo': { target: '<other_url>' } } } }
|
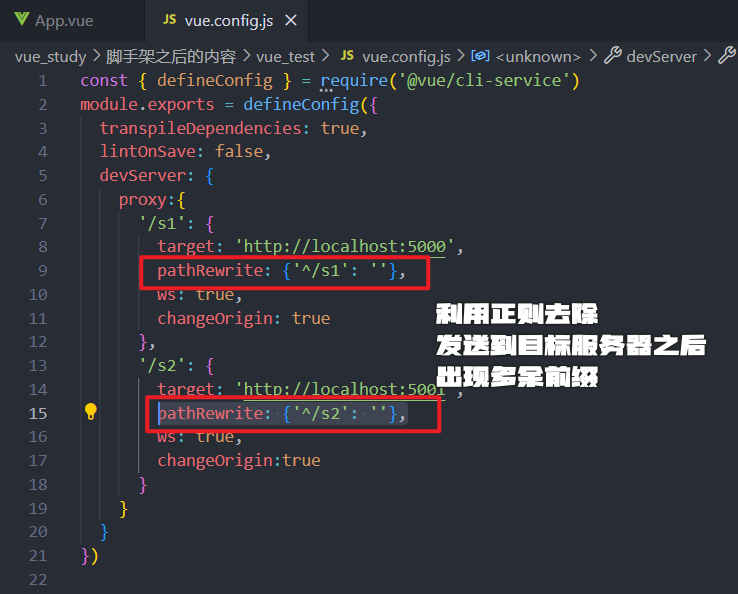
笔记&练习代码
App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| <template> <div> <button @click="getStusInfo">接收学生信息</button> <button @click="getCarsInfo">接收汽车信息</button> </div> </template>
<script> import axios from 'axios' export default { name:'App', data() { return { name:'zepore' } }, methods: { getStusInfo(){ axios.get('http://localhost:8080/s1/students').then( response=>{ console.log('请求成功:', response.data); }, error=>{ console.log('请求失败:', error.message); } ) }, getCarsInfo(){ axios.get('http://localhost:8080/s2/cars').then( response => { console.log('请求成功:' , response.data); }, error => { console.log('请求失败:' , error.message); } ) } } } </script>
|
vue.config.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| const { defineConfig } = require('@vue/cli-service') module.exports = defineConfig({ transpileDependencies: true, lintOnSave: false, devServer: { proxy:{ '/s1': { target: 'http://localhost:5000', pathRewrite: {'^/s1': ''}, ws: true, changeOrigin: true }, '/s2': { target: 'http://localhost:5001', pathRewrite: {'^/s2': ''}, ws: true, changeOrigin:true } } } })
|
GitHub搜索案例
为何不放asset?
引入静态资源,会进行严格检查,没有的字体文件会报错
技术难点/坑
使用模板进行拼接链接
https://api.github.com/search/users?q=${this.inputVal}
里的 ${this.inputVal}
代码练习
MyHeader.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| <template> <section class="jumbotron"> <h3 class="jumbotron-heading">Search Github Users</h3> <div> <input type="text" placeholder="enter the name you search" v-model="inputVal" @keyup.enter="searchViaLogin" /> <button @click="searchViaLogin">Search</button> </div> </section> </template>
<script> import axios from 'axios' export default { name:'MyHeader', data() { return { inputVal:'', } }, methods: { searchViaLogin(){ this.$bus.$emit('userList',{isFirst:false, isLoading:true, users:[], errMsg:''}) axios.get(`https://api.github.com/search/users?q=${this.inputVal}`).then( receive =>{ // console.log(receive.data); this.$bus.$emit('userList',{isLoading:false, users:receive.data.items, errMsg:''}) }, error => { console.log(error.message); this.$bus.$emit('userList',{isLoading:false, users:[], errMsg:error.message}) } ) } } } </script>
|
MyList.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73
| <template> <div class="row"> <!-- 发送成功之后 --> <div class="card" v-for="user in info.users" :key="user.login"> <a :href="user.html_url" target="_blank"> <img :src="user.avatar_url" style='width: 100px'/> </a> <p class="card-text">{{user.login}}</p> </div> <!-- 第一次展示欢迎词 --> <div v-show="info.isFirst"> <h1>欢迎使用</h1> </div> <!-- 搜索过程展示加载中 --> <div v-show="info.isLoading"> <h1>加载中...</h1> </div> <!-- 搜索错误展示错误信息 --> <div v-show="info.errMsg"> <h1>{{info.errMsg}}</h1> </div> </div> </template>
<script> export default { name: 'MyList', data() { return { info: { isFirst:true, isLoading:false, users:[], errMsg:'' } } }, mounted() { this.$bus.$on('userList', (info)=>{ console.log('List这边收到了',info); // this.users = users; this.info = info }) }, } </script>
<style> .album { min-height: 50rem; /* Can be removed; just added for demo purposes */ padding-top: 3rem; padding-bottom: 3rem; background-color: #f7f7f7; }
.card { float: left; width: 33.333%; padding: .75rem; margin-bottom: 2rem; border: 1px solid #efefef; text-align: center; }
.card > img { margin-bottom: .75rem; border-radius: 100px; }
.card-text { font-size: 85%; } </style>
|
App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <template> <div class="container"> <MyHeader /> <MyList/> </div> </template>
<script> import MyHeader from './components/MyHeader.vue' import MyList from './components/MyList.vue' export default { name:'App', data() { return { name:'zepore' } }, components:{MyHeader,MyList} } </script>
|