2月20到21这两天的项目维护
关于Git
公司用的是云服务器搭建的GitLab,我遇到一个困扰挺久的问题,就是推送代码上去的时候经常出现报错:
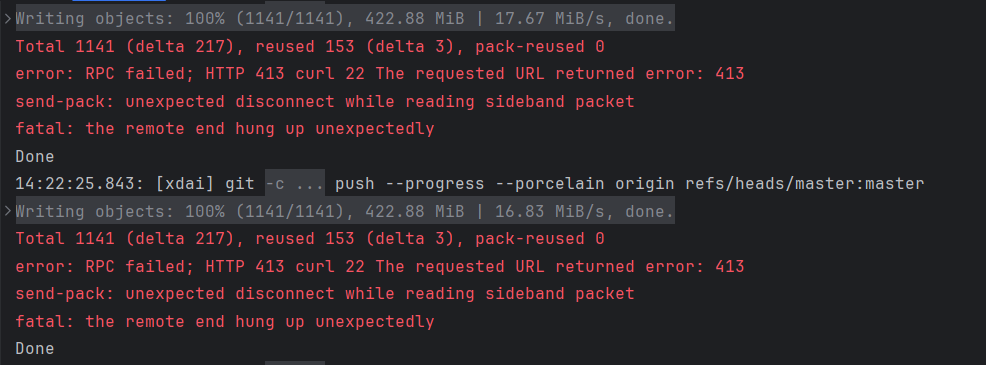
从网上寻找答案得知
413错误码——http请求实体太大
这要么是上传时我们这边的git有上传大小的限制,要么就是服务器那边做了限制,尝试修改本地Git上传大小限制无果之后原因就是后者了,而且GitLab大概率使用的是nginx搭建的,出于与搭建者并不熟悉的关系,只能不使用http方式上传,改用ssh。
1 2 3 4 5 6
| 原因分析:上传文件太大,超过了最大限制 解决方法:nginx传输限制:(nginx.conf) client_max_body_size 400M; git传输限制: git config --global http.postBuffer 524288000 git config --global https.postBuffer 524288000
|
关于SSH配置,网上教程很多,无非就是生成Token,然后绑定到GitLab。
关于日志的打印
mybatis日志的打印一般会放在appplication.yml
文件里面,而打印级别一般没放在一起,而是放在-dev
/ -prod
文件(具体看application.xml
的${spring.profiles.active}
)里面的logging项里面
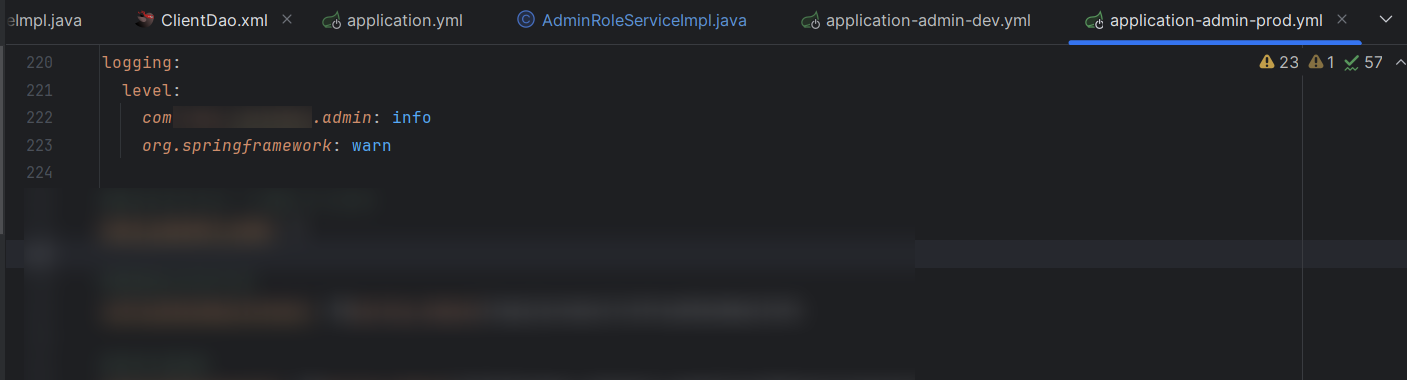
关于打包
公司的项目不同于我在学校接触到的小项目,代码通过maven打包之后生成的是一个zip文件,里面包含了配置文件和依赖包,可以直接一键部署到服务器上,特别高效。
完整的pom文件build配置脱敏如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90
| <build> <finalName>${project.artifactId}</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> <encoding>UTF-8</encoding> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <configuration> <archive> <manifest> <mainClass>XXXApplication</mainClass> <useUniqueVersions>false</useUniqueVersions> <addClasspath>true</addClasspath> <classpathPrefix>lib/</classpathPrefix> </manifest> <manifestEntries> <Class-Path>config/</Class-Path> </manifestEntries> </archive> <classesDirectory> </classesDirectory> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-dependency-plugin</artifactId> <executions> <execution> <id>copy</id> <phase>package</phase> <goals> <goal>copy-dependencies</goal> </goals> <configuration> <outputDirectory> ${project.build.directory}/lib </outputDirectory> </configuration> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-resources-plugin</artifactId> <configuration> <encoding>UTF-8</encoding> <nonFilteredFileExtensions> <nonFilteredFileExtension>xlsx</nonFilteredFileExtension> <nonFilteredFileExtension>xls</nonFilteredFileExtension> <nonFilteredFileExtension>p12</nonFilteredFileExtension> </nonFilteredFileExtensions> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-assembly-plugin</artifactId> <configuration> <descriptors> <descriptor>src/main/assembly/assembly.xml</descriptor> </descriptors> </configuration> <executions> <execution> <id>make-assembly</id> <phase>package</phase> <goals> <goal>single</goal> </goals> </execution> </executions> </plugin> </plugins> </build>
|
关于数据库出现0000-0000…日期错误
报错:MySQL:[Err] 1292 - Incorrect datetime value: ‘0000-00-00 00:00:00‘ for column ‘CREATE_TIME‘
在做数据迁移的时候出现了上面这个问题,应该不同的MySQL对日期初始值的设置不一样导致的,为了兼容这个问题,要把全局的sql_mode默认的NO_ZERO_DATE、NO_ZERO_IN_DATE去掉,参考博文
然后还要记得降级新部署的MySQL版本到5.7及以下
同时如果是Java项目,使用到jdbc时要在数据库url加上&zeroDateTimeBehavior=CONVERT_TO_NULL

结合 Redis 对文件判重的一个实现
2024.02.23:今天学习到一个专业术语来描述这个效果:幂等
前面对导入文件进行了优化,项目管理就提了一个问题:

整个系统上传文件都限制在2M以内,调了接口就算离开页面,只要上传结束并不会对导入的数据造成影响,后端会一直处理直到数据全部导入。因此,应该规避的就是用户重复上传。结合自身能力,我选择利用Redis来实现这一简单的功能。
我的思路是获取收到的文件的哈希值,判断该哈希是否存在于缓存中,如果是新哈希,就将其存储到Reids,缓存时间设置为6分钟,代码实现如下:
Redis业务层主要有三个功能:判断哈希值是否已经存在、添加文件哈希进缓存、设置缓存时间
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| import java.util.concurrent.TimeUnit;
@Service public class FileHashServiceImpl implements FileHashService {
private static final String FILE_HASH_KEY = "file_hashes";
@Autowired private RedisTemplate<String, String> redisTemplate;
public boolean isFileHashExists(String hash) { return Boolean.TRUE.equals(redisTemplate.opsForSet().isMember(FILE_HASH_KEY, hash)); }
public void addFileHash(String hash) { redisTemplate.opsForSet().add(FILE_HASH_KEY, hash); }
public void setFileHashExpiration(String hash, long timeout, TimeUnit unit) { redisTemplate.expire(FILE_HASH_KEY, timeout, unit); } }
|
控制层
1 2 3 4 5 6 7 8
| String sha256Hex = DigestUtil.sha256Hex(file.getInputStream()); if(!fileHashService.isFileHashExists(sha256Hex)) { fileHashService.addFileHash(sha256Hex); fileHashService.setFileHashExpiration(sha256Hex, 6, TimeUnit.MINUTES); } else { return new Result("0", "请勿重复上传"); }
|